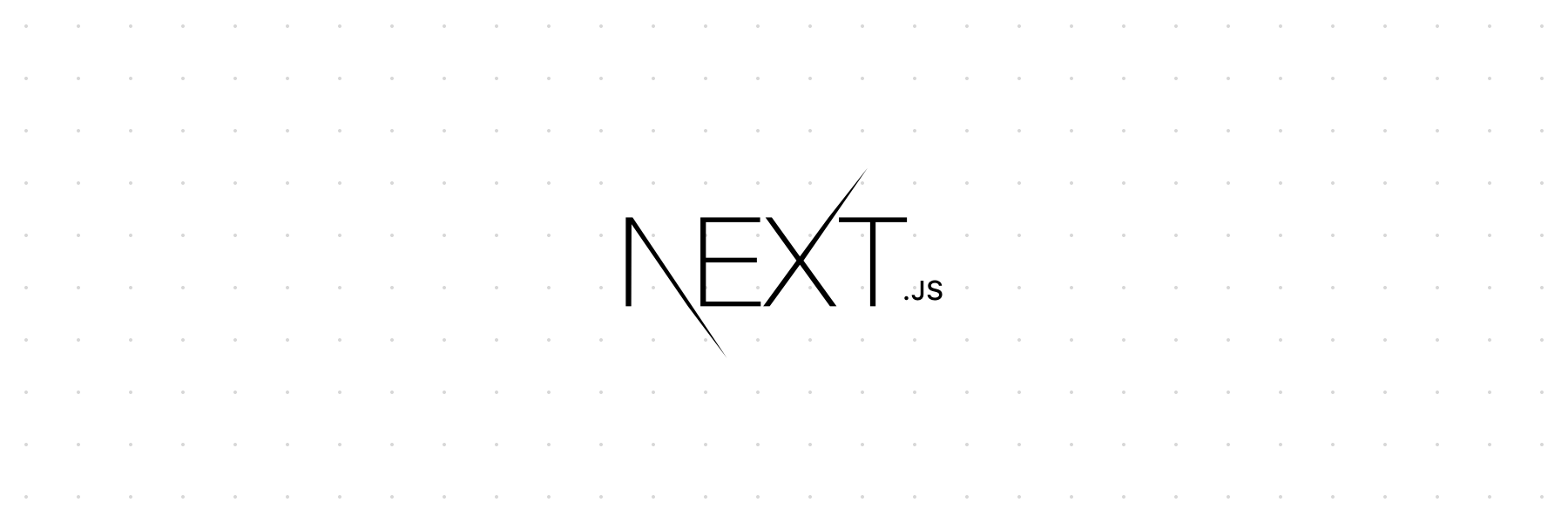
What is Next.js? (Sample Project) (Outdated)
SSR (Server Side Rendering): Before libraries like Vue, React, Backbone became popular, this rendering method was commonly preferred. In SSR, the server fills all the necessary content and sends it to the client. As a result, when the client downloads the HTML file, all the data is already available to the client.
CSR (Client Side Rendering): In this rendering method, unlike SSR, the server sends an empty HTML file and JavaScript files to the client to fill the content. From this point on, routing and content filling processes are performed by the client. The blank HTML page received is populated by the client and served.
Open Graph: Open Graph was first introduced by Facebook in 2010 to facilitate information exchange between users, websites, and Facebook. It is used to ensure that website pages can be understood and shared correctly by Facebook. Open Graph meta tags are used to achieve this. Twitter also has similar meta tags to Open Graph.
Next.js
Although Google and modern search engines claim that they can crawl SPA (Single Page Application) without issues, there are cases where they may struggle to crawl pages correctly. In such cases, to make your React applications more SEO-friendly, you can either utilize paid services like prerender.io or develop applications using React with SSR (Server-Side Rendering) using frameworks like Next.js.
In the rest of the article, I will continue by explaining some advantages provided by Next.js through an example Next.js project.
Installation:
There are two options for installing Next.js: you can either use npx or directly add the next, react, and react-dom packages using npm. This article will explain the installation using npx.
To install the project:
npx create-next-app
To run the project:
npm run dev
When you run the project, a single-page website will be served at localhost:3000. If you look into the project files, you will see an index page under the pages folder. When you explore the other files, you will notice that there is no separate file explicitly defining the routes as you would typically do in a React.js project. This is where Next.js's File-System Routing feature comes into play.
Each .js file you open under the pages folder serves as a route as well. When you create a file named articles.js under the pages folder, for example, accessing localhost:3000/articles will directly render the component defined in the articles.js file. This is the power of Next.js's File-System Routing feature, where the file structure itself determines the routing behavior of the application.
Head:
With Next.js, you can use the Head component, which is one of the most important components that comes with Next.js, to render and serve meta tags, including Open Graph tags, on the server.
While solutions like Helmet can achieve similar functionality in React, the HTML heads prepared with Helmet, unfortunately, are not visible to social platforms. On the other hand, Next Head renders your HTML head on the server and serves it to the client, ensuring that there are no SEO issues. All you need to do is encapsulate your meta tags within the Head component. You can set up this structure separately for each page or add the tags by default to the top-level component in your component tree.
If you need to fetch data from an API, in React, you have options like Axios and Fetch. However, none of these options, by nature of React, perform the request before the HTML is generated.
The requests are made after the page has loaded, resulting in initially seeing an empty HTML page. In the case of Next.js, since it runs on the server-side with Node.js, we have the ability to make the specified requests before serving the HTML. This is where the "getInitialProps" feature provided by Next.js comes in.
First, we need to add the "isomorphic-unfetch" library to our project:
npm install — save isomorphic-unfetch
For our sample project, we will use a simple news API provided by https://newsapi.org/. You can easily create your own API key on their website, https://newsapi.org/.
Articles.getInitialProps = async function() {
const apiPath = "https://newsapi.org/v2/";
const res = await fetch(`${apiPath}everything?q=bitcoin&from=2019-11-02&sortBy=publishedAt&apiKey=<ApiKey>`);
const data = await res.json();
return {
articles: data.articles
};
};
We are adding the static and asynchronous code block to our articles page.
The sole purpose of this function is to fetch data from an API and return it as a prop to the specified component. We will use the data returned as a prop to display it within the Articles component, listing the information.
import React from 'react'
import Head from 'next/head'
import Nav from '../components/nav'
import fetch from 'isomorphic-unfetch'
const Articles = (props) => {
const {articles} = props;
return(
<div>
<Head>
<title>Articles</title>
<meta property="og:title" content="Next.js Articles" />
<meta property="og:image" content="/next.png" />
<meta property="og:type" content="video.movie" />
<meta property="og:description" content="Lorem ipsum dolares" />
<meta property="og:url" content="www.test.url" />
<link rel="icon" href="/favicon.ico" />
</Head>
<Nav />
<h1 className="page-title">Articles</h1>
<div className='article-list'>
{articles.map((article)=>(
<div className='article-list__article'>
<h1>{article.title}</h1>
<p>{article.description}</p>
<button>Read More</button>
</div>
))}
</div>
</div>)
}
Articles.getInitialProps = async function() {
const apiPath = "https://newsapi.org/v2/";
const res = await fetch(`${apiPath}everything?q=bitcoin&from=2019-11-02&sortBy=publishedAt&apiKey=<ApiKey>`);
const data = await res.json();
return {
articles: data.articles
};
};
export default Articles;
When we view the page source by accessing localhost:3000/articles, we will see that all the data we requested from the API and the meta tags are rendered in the HTML. In this case, we have solved many of the SEO issues that arise with SPAs (Single Page Applications).
Deployment Proccess:
npm run build
Indeed, when you build a React application, you get static HTML files under the build folder. However, in Next.js, the situation is a bit different. When you run the build command in a Next.js project, Next.js lists the built files under the .next folder.
Unlike the static .html files under the build folder in React, you won't be able to open the Next.js project's files in the same way due to server-side rendering (SSR) happening in Next.js. To serve the project, it's necessary to have a Node server running in the background. For deployment, you can use services like PM2 to manage the server and keep the application running.
npm run start
Conclusion:
In this article, I have only touched upon a few advantages provided by Next.js. Apart from these features, Next.js offers many other capabilities such as automatic AMP page generation and creating fast API services.
With Next.js, you can quickly set up a project without the need for complex configurations or writing custom routes, thanks to the ease of use provided by the "npx" command. When we look at Google Lighthouse and other testing tools, we can see that Next.js receives high scores in performance and other areas.